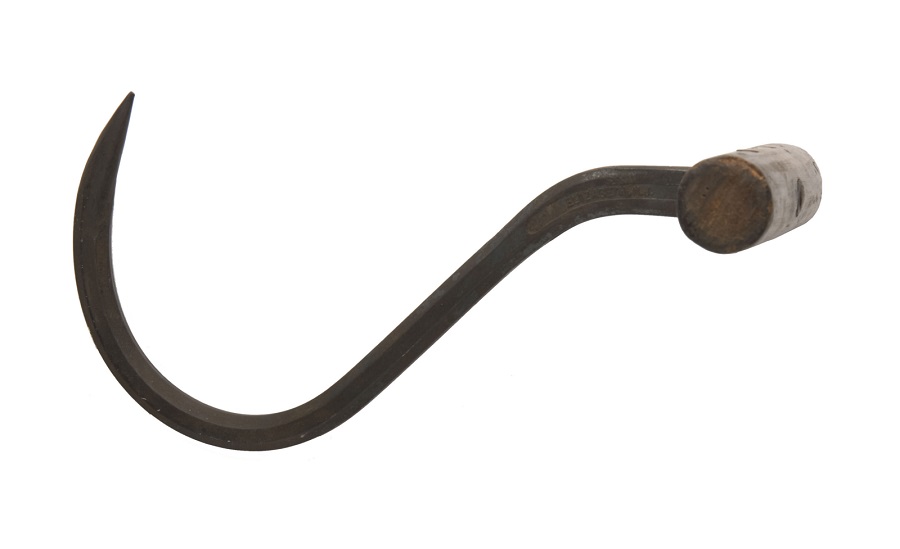
I am working on a UI Library project. Here is my set of Git Hooks that I have added to my project. These automated scripts run on the user’s local development environment prior to getting pushed up to the remote Git repository. The scripts run quickly and help validate that initial code quality checks are working before making them available on a shared repo.
module.exports = {
hooks: {
'commit-msg': 'commitlint -E HUSKY_GIT_PARAMS',
'pre-commit': 'lint-staged && ng lint && npm run lint:styles',
'pre-push': 'npm run test:projects:headless && npm run e2e:projects:headless && npm run build:projects:prod',
'post-merge': 'post-npm-install',
'post-rebase': 'post-npm-install'
}
};
Here’s a description of what this code means:
The various keys in the hooks object represent the different triggers for when to run each of the NPM commands on the code that is committed.
First, we run the pre-commit hook when we commit code into our local dev Git branch where we lint and format our code files by running a lint-staged command that runs Prettier, tslint, and stylelint on all pre-commit files. The hook then runs ng lint and style:styles commands on all other files in the project to check the styles on all .ts and .scss files, respectively.
Second, the commit-msg hook will also run during our code commit step. Here we run the commitlint command which will lint our commit message so that it conforms to conventional Git commits standards.
Third, when the developer finally pushes their commits up to a Git remote, a pre-push hook will trigger. This Git hook runs unit tests, end-to-end tests, and production build locally on the developer’s machine. If any of these automation fail, then the code is not pushed up to the remote repository. Better to know in advance that your code is not well tested while developing locally than to be told later by a CI process.
Finally, whenever a local dev branch is rebased or upstream commits are merged, then either post-rebase or post-merge hook will trigger. The hooks execute the post-npm-install command that automatically runs “npm install” if the package.json file has changed. This allows the developers to have all libraries used in the project up-to-date.
Information about Git Hooks: https://www.git-scm.com/docs/githooks
3rd party Node packages: